Blog
During the course of the day I encounter lots of challenges, some of which take minutes to solve and others that take much longer. My goal for this section is to keep track of these challenges and their solutions. I will turn the longer ones into articles, while the shorter ones will stay as blog entries. You can expect topics to range from very specific programming challenges to broader topics like life.
Below is a list of the recent blog entries. You can also browse the blog by using the tags on the right side, or if you know what you are looking for then you can use the search box at the top right.
I recently had the need to output a SELECT HTML element with a list of states. My initial approach was simple where I used <xsl:copy>
…
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:custom="com.thebitguru" exclude-result-prefixes="custom">
<xsl:output method="html" omit-xml-declaration="yes"/>
<xsl:strip-space elements="*" />
<custom:states>
<option value="AL">Alabama</option>
<!-- ... -->
<option value="WI">Wisconsin</option>
<option value="WY">Wyoming</option>
</custom:states>
<xsl:template match="option">
<xsl:copy>
<xsl:if test="@value = $val">
<xsl:attribute name="selected">selected</xsl:attribute>
</xsl:if>
<xsl:attribute name="value"><xsl:value-of select="@value" /></xsl:attribute>
<xsl:value-of select="." />
</xsl:copy>
</xsl:template>
<xsl:template match="*">
<label for="state">* State:</label>
<select name="state" id="state">
<xsl:apply-templates select="document('')/*/custom:states/option" />
</select>
</xsl:template>
</xsl:stylesheet>
|
The problem with this approach was that the option
tags were printing the xml namespaces, even with the exclude-result-prefixes="custom"
specified (because that attribute does not apply to <xsl:copy>
).
|
<option value="AL" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:custom="com.thebitguru">Alabama</option>
...
<option value="WI" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:custom="com.thebitguru">Wisconsin</option>
<option value="WY" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:custom="com.thebitguru">Wyoming</option>
|
I didn’t want these namespaces in the output so after some searching and reading through the docs I decided to create new elements using the <xsl:element>
tags instead, especially since the tag that I was copying was very small.
|
<xsl:template match="option">
<xsl:element name="option">
<xsl:if test="@value = $val">
<xsl:attribute name="selected">selected</xsl:attribute>
</xsl:if>
<xsl:attribute name="value"><xsl:value-of select="@value" /></xsl:attribute>
<xsl:value-of select="." />
</xsl:element>
</xsl:template>
|
There are other ways to accomplish the same thing, e.g. copy-namespaces in XSLT 2.0, but in my case I was stuck with XSLT 1.0.
I recently had the need to cleanup a long diff file and move certain lines to the end of the file for further analysis. The diff file that I got was something like the following, only much longer (produced using diff -qr xxx yyy
).
Files xxx/abc1 and yyy/abc1 differ
Only in xxx: cde1
Files xxx/abc3 and yyy/abc3 differ
Files xxx/abc4 and yyy/abc4 differ
Only in xxx: cde2
Only in xxx: cde5
Files xxx/abc5 and yyy/abc5 differ
Only in xxx: cde3
I didn’t care as much about the “Only…” lines, but the files that differed needed more attention. To accomplish this I wanted to get this file in the following format.
Only in xxx: cde1
Only in xxx: cde2
Only in xxx: cde5
Only in xxx: cde3
Files xxx/abc1 and yyy/abc1 differ
Files xxx/abc3 and yyy/abc3 differ
Files xxx/abc4 and yyy/abc4 differ
Files xxx/abc5 and yyy/abc5 differ
I could go in and copy and paste all the lines that matched, but that would be a lot of manual work. So, I looked around for a minute and came up with the following command.
:%g/^Files/m$
Vim is awesome!
My original patch possibly worked only with OS X 10.6.5. Last night I figured out a better way to apply the patch without relying on a pre-supplied diff. What this means is that starting with version 0.7 the patch should work with pretty much all versions.
If you are interested, check it out on the project page.
I just published a new patch for OS X, below is a description of the patch. Check it out.
This is a patch for removing the default OS X behavior of always starting iTunes when the play button on the keyboard is pressed. This feature can be useful for a lot of users, but it can also be annoying if you are using VLC or other similar programs that support the media keys.
The Patch script will patch the Remote Control Daemon to prevent it from starting iTunes whenever you press the play button on the keyboard or an external remote control. This will only prevent iTunes from starting, all other functions (like play/pause while iTunes is running) will continue to work as before.
Lastly, this program will backup the original file in case if you would like to restore the original functionality.
For more information please visit the Play Button iTunes Patch page.
One thing I have missed in OS X is a powerful media player like MediaMonkey. iTunes gets a good part of the job done, but doesn’t have everything that I need. Plus, I hate the fact that it doesn’t display the ratings that I have on the files from my Windows machine. So, I have been exploring my options and one possibility is Songbird. It it better, but still lacks in some places (once again, ratings). I am still playing around with Songbird because, compared to iTunes, it is a lot easier to create add-ins for Songbird.
The first thing that I noticed missing from Songbird was the ability to delete songs that are not “managed” by Songbird. This is pretty much how iTunes behaves as well. I did not like this because I like control. If I added a file to a playlist then I would also like to have the ability to delete it from both the playlist and the file system.
So, instead of using the prescribed “1-start rating” to denote deletions I decided to explore Songbird extension development. I am glad to report that I was able to figure out the framework and actually just published an extension in the official repository to do just that: Delete from Disk. If you are a Songbird user looking for this functionality then you don’t need to look any further. Browse to the extensions page in Songbird and search for Delete from Disk.
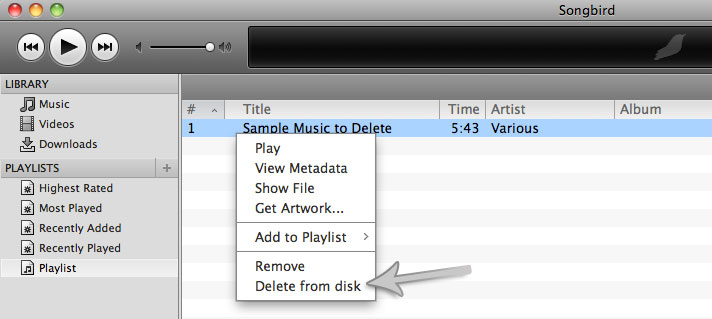
Now let’s figure out what we can do about the ratings…! I also wish that someone will fix the Apple media key support extension.
Lately I have noticed that I kept getting a 400 bad request when doing django development (i.e. using runserver) and browsing through Google Chrome. It was only Chrome that was behaving this way because Firefox would browse without generating any of these errors on the server side.
1
2
3
4
|
[09/Nov/2010 00:31:53] "GET /acq/ HTTP/1.1" 200 1393
[09/Nov/2010 00:31:53] code 400, message Bad request syntax ('\x16\x03\x00...')
?????????...." 400 -???8 .....?????
[09/Nov/2010 00:33:39] "GET /acq/ HTTP/1.1" 200 1393
|
I finally took a few minutes to debug. After clearing the cache and browsing data as suggested by many sites and having no luck I started disabling all of the extensions that I had installed. Guess what? The error disappeared.
This issue was being caused by the Secure Sites extension which lets you use secure versions of the site if one is available. In order to figure out whether a secure version is available it does an extra request, and it is this extra request that was causing the problem.
What’s the solution? I went into the options for this extension and added localhost to “Assumed no secure version exists” and the error disappeared.
I just published an updated version of the Wake on LAN (WoL) client for webOS (Palm Pre and Pixi). The latest version supports specifying a custom hostname and port, which means that now you can send WoL packages from to a remote network.
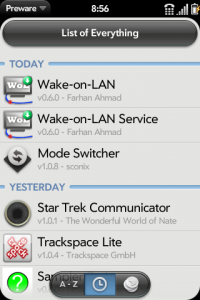
James Knowles (please see the about screen for contact info) contributed the initial UI changes for specifying a hostname. I added some more information and added the ability to specify a custom port (which required a service update).
To get these changes please update to/install the latest version of the service and UI using Preware.
…that the monitors at the Chicago O’Hare airport are running Adobe Flash! 🙂
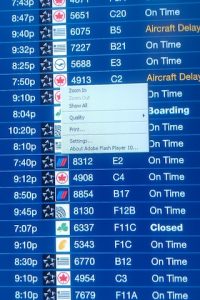
MediaMonkey (MM) is my music player of choice on Windows. I have a few global hotkeys setup (for instance WIN+ALT+B for next track), but after an upgrade a few months ago (probably starting with 3.0) I noticed that my shortcuts started sending the shortcut keys to the active applications. So, for instance, while in Outlook if I press WIN+ALT+B, MM will go to the next track, but in addition Outlook will switch views because ALT+B is a shortcut in Outlook.
After a quick post online I found the solution that I was looking for. Basically, I had to edit the MediaMonkey.ini and add “PreferLLKeysHook=1” to the “[options]” section. MediaMonkey.ini will be located in different places based on the operating system, you can find the details in this knowledge base article. Add “PreferLLKeysHook=1” to the “[options]” section in there and restart MM. From there on the shortcut keys will only be interpreted by MM and will not be forwarded to the active application.
Lately I have been playing around with Objective-C development on mac. As I was working through a tutorial I kept getting the following error for a calculated read-only property.
[<NSManagedObject 0x2000c3c20> valueForUndefinedKey:]: the entity Expense is not key value coding-compliant for the key "filename".
Below is the definition of the class.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
// Expense.h
#import <Cocoa/Cocoa.h>
@interface Expense : NSManagedObject {}
@property (readonly) NSString* filename;
@end
// Expense.m
#import "Expense.h"
@implementation Expense
- (NSString *) filename {
return @"TestingPath";
}
@end
|
The class definition is OK so the error didn’t make sense. After some troubleshooting I found out what was causing this. Since this was my first application I didn’t know that I had to tell the model what class it should be using, and, unfortunately, the book that I was using didn’t mention it either (or maybe I missed it).
Anyways, if you get this error then make sure that you have associated the class with the model. To do this you have to specify the class name under “Class” in the model definition (see screenshot below).
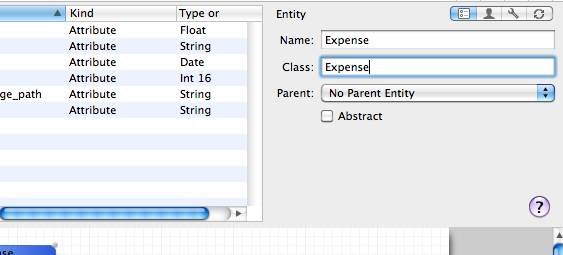